Blog Details
Step-by-Step Guide to Integrating Stripe Payments in Laravel
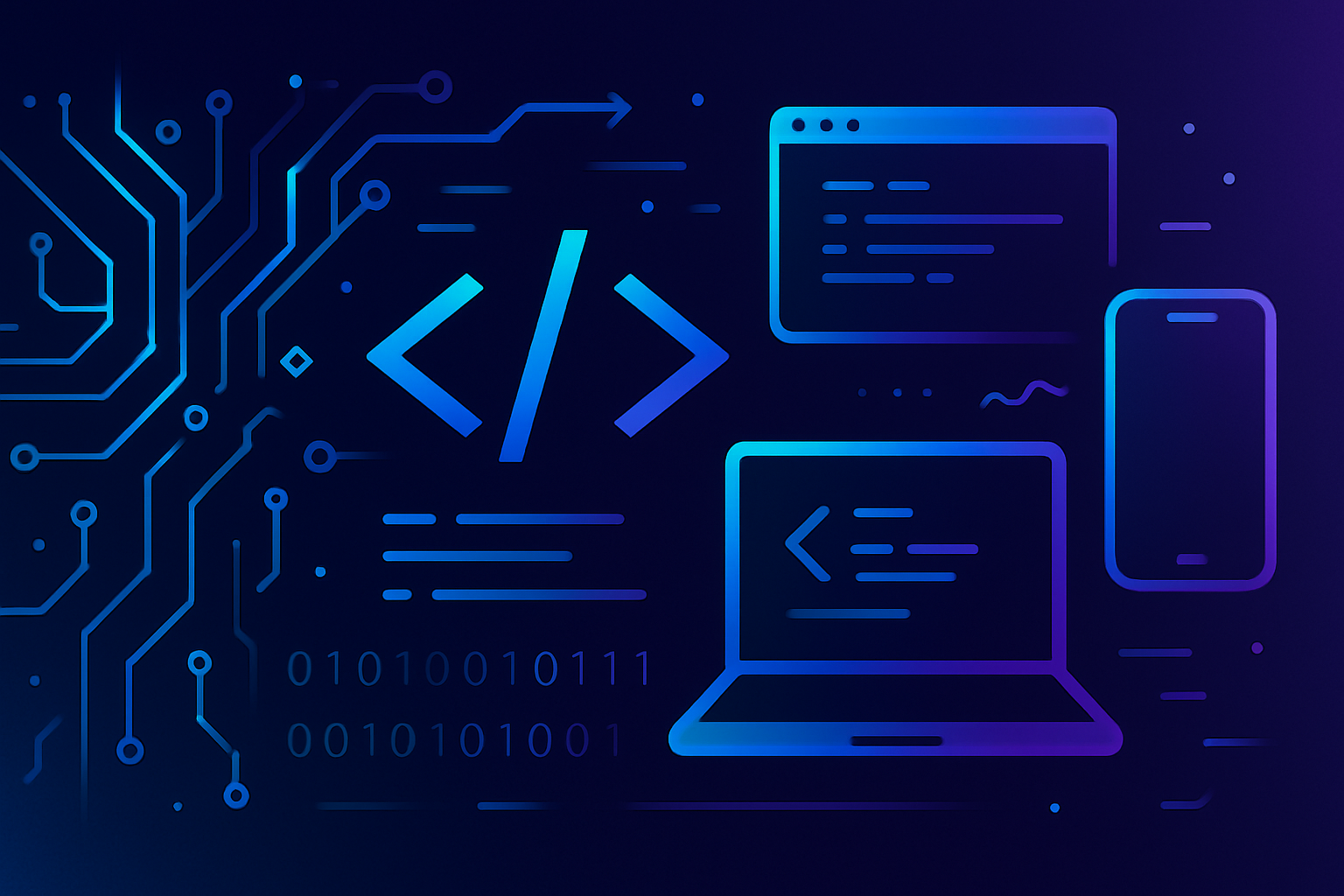
Introduction:
Laravel is a popular PHP web framework that provides a comprehensive suite of tools and features for building robust web applications. One of the most essential features that web applications need is a payment gateway, which allows users to make payments securely and efficiently. Stripe is one of the most popular payment gateways available, and integrating it with Laravel can be a straightforward process. In this article, we will take a look at how to integrate Laravel with Stripe.
Step 1: Create a Stripe Account
Before we start integrating Stripe with Laravel, you will need to create a Stripe account. You can sign up for a free account on the Stripe website. Once you have signed up, you will need to verify your email address and activate your account.
Step 2: Install the Stripe Package for Laravel
The first step in integrating Laravel with Stripe is to install the Stripe package for Laravel. You can do this using Composer, which is a PHP package manager. Open up your terminal and navigate to your Laravel project directory. Then, run the following command:
composer require stripe/stripe-php
This command will install the Stripe PHP library, which is required for the integration.
Step 3: Configure Stripe Keys
To use the Stripe API, you will need to configure your Stripe keys in your Laravel application. Open up the .env
file in your Laravel project directory and add the following lines:
STRIPE_KEY=
STRIPE_SECRET=
Replace and with your actual Stripe API keys.
Step 4: Create a Stripe Charge
Now that you have installed the Stripe package for Laravel and configured your Stripe keys, you can start using the Stripe API to process payments. In this example, we will create a simple form that allows users to make a payment.
Create a new Laravel route in your routes/web.php
file, like so:
Route::get('/charge', function () {
return view('charge');
});
This route will display a form that allows users to enter their payment details.
Next, create a new view file called charge.blade.php
in the resources/views
directory. This file should contain a form that allows users to enter their payment details. Here's an example:
{{ csrf_field() }}
Amount (in cents):
Email:
Credit or debit card
Submit Payment
This form includes fields for the payment amount, the user’s email address, and a credit card input field. The Stripe JS library is also included at the bottom of the file, which is required for handling credit card payments.
Next, create a new Laravel route that handles the form submission:
Route::post('/charge', function (Request $request) {
// Set your Stripe API key.
\Stripe\Stripe::setApiKey(env('STRIPE_SECRET'));
// Get the payment amount and email address from the form.
$amount = $request->input('amount') * 100;
$email = $request->input('email');
// Create a new Stripe customer.
$customer = \Stripe\Customer::create([
'email' => $email,
'source' => $request->input('stripeToken'),
]);
// Create a new Stripe charge.
$charge = \Stripe\Charge::create([
'customer' => $customer->id,
'amount' => $amount,
'currency' => 'usd',
]);
// Display a success message to the user.
return 'Payment successful!';
});
This route retrieves the payment amount and email address from the form, creates a new Stripe customer, and charges the customer’s credit card.
Step 5: Test the Integration
To test the integration, start your Laravel development server by running the following command in your terminal:
php artisan serve
Then, open up your web browser and navigate to `http://localhost:8000/charge`. You should see the payment form that you created earlier.
Enter a payment amount, email address, and credit card details, and click the “Submit Payment” button.
If everything is set up correctly, you should see a “Payment successful!” message displayed on the screen. You can also check your Stripe dashboard to verify that the payment was processed successfully.
Conclusion
Integrating Laravel with Stripe is a straightforward process that can be done in just a few steps. By following the steps outlined in this article, you can quickly and easily add payment processing to your Laravel web application. With the power of Laravel and the flexibility of Stripe, you can create a robust and secure payment system that meets the needs of your users.