Blog Details
How to Create Custom Helpers in Laravel
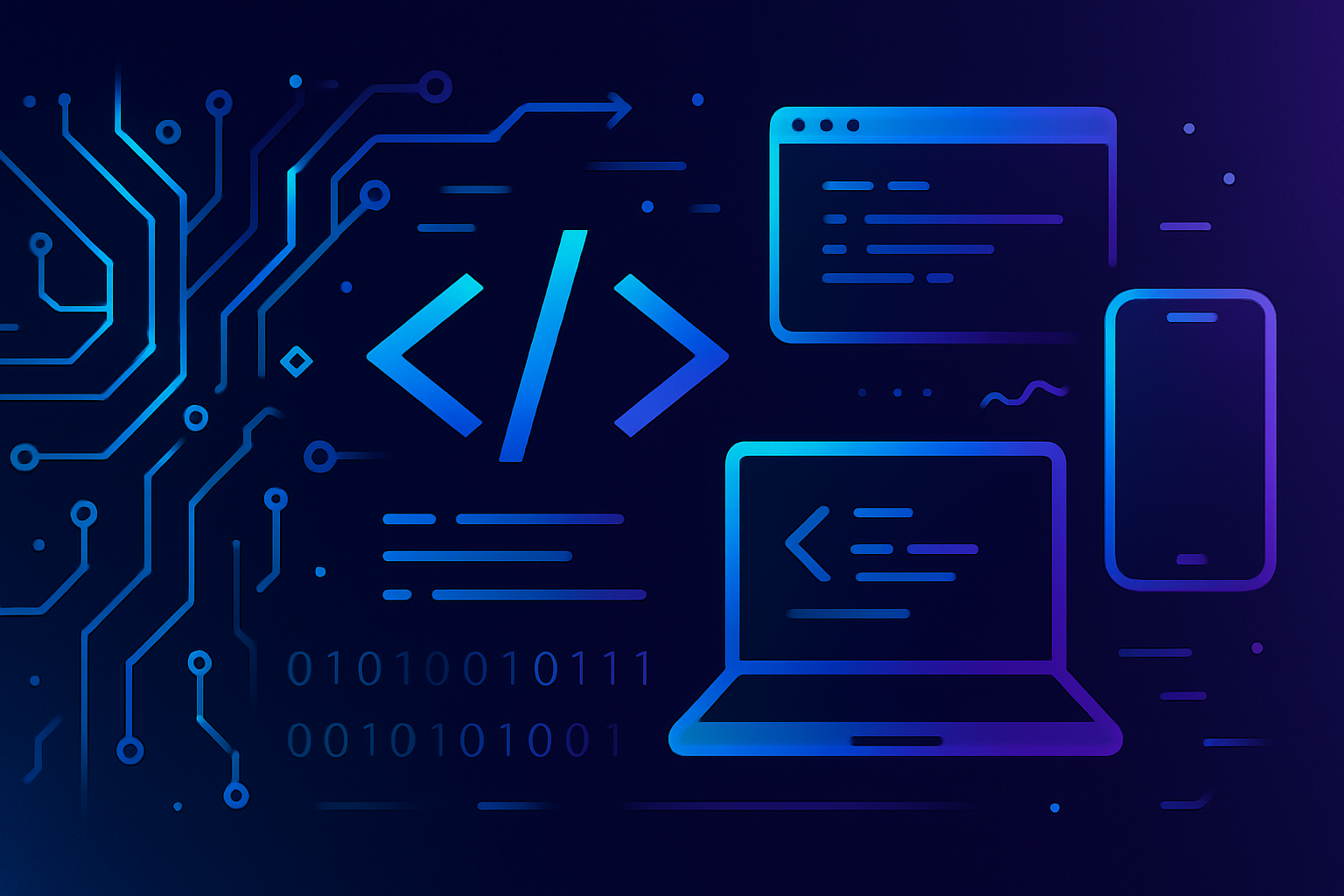
Laravel is a powerful and flexible PHP framework widely used for developing modern web applications. One of the many features that make Laravel so user-friendly is the ability to create custom helper functions. These helper functions allow you to add reusable code snippets that can be accessed throughout your application. In this blog, we will discuss how to create a helper in Laravel 10, ensuring that your Laravel development process becomes even more efficient.
What is a Laravel Helper?
A Laravel helper is a custom function that you can call anywhere in your Laravel application. These functions are typically used for tasks that you need to perform repeatedly, such as formatting dates, manipulating strings, or handling arrays. By centralizing these tasks in helper functions, you can keep your code clean, organized, and easy to maintain.
Steps to Create a Helper in Laravel 10
1. Create the Helper File
First, you need to create a new PHP file where you will define your helper functions. A common convention is to create a helpers.php file within the app directory.
cd app
touch helpers.php
2. Define Your Helper Functions
Open the newly created helpers.php file and start defining your helper functions. For example, let's create a simple helper function that formats dates.
format($format);
}
}
3. Autoload the Helper File
To make your helper functions available throughout your Laravel application, you need to autoload the helpers.php file. Open the composer.json file located at the root of your Laravel project.
Add the helpers.php file to the autoload section.
"autoload": {
"files": [
"app/helpers.php"
]
}
4. Update Composer
After modifying the composer.json file, you need to run composer dump-autoload to regenerate the autoload files.
composer dump-autoload
5. Use Your Helper Functions
Now, you can use your custom helper functions anywhere in your Laravel application. For instance, you can use the format_date function in a controller, view, or any other part of your application.
// In a Controller
public function show($id) {
$date = '2024-05-26';
$formattedDate = format_date($date, 'd/m/Y');
return view('show', compact('formattedDate'));
}
Best Practices for Creating Laravel Helpers
- Namespace and Organize: If you have a large number of helper functions, consider organizing them into different files or namespaces for better readability and maintenance.
- Document Your Helpers: Always document your helper functions to provide clear instructions on their usage and parameters.
- Avoid Global Helpers: Where possible, avoid creating global helper functions that can cause conflicts. Use namespacing or class-based helpers instead.
Conclusion
Creating custom helper functions in Laravel 10 can significantly streamline your development process by providing reusable and accessible code snippets. By following the steps outlined in this blog, you can easily create and manage your own Laravel helpers. This will not only make your codebase cleaner but also improve your productivity as a developer.
By leveraging the power of Laravel helpers, you can ensure that your application remains organized and easy to maintain, allowing you to focus on building great features and delivering value to your users. Happy coding!