Blog Details
Mastering Laravel Eloquent and Resource Controllers
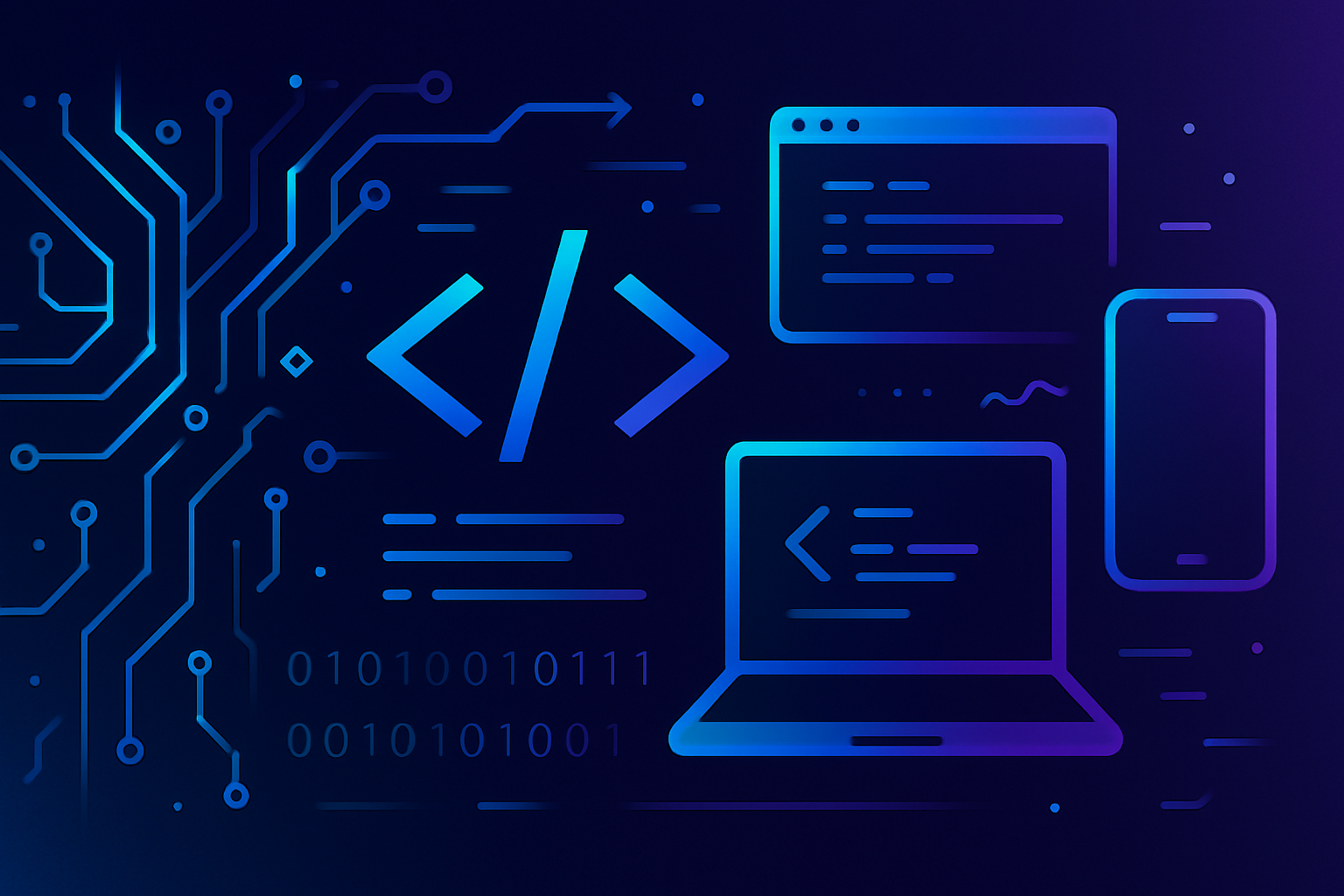
Introduction:
Welcome to a comprehensive guide on mastering Laravel Eloquent and Resource Controllers! In this tutorial, we'll explore Laravel's powerful ORM - Eloquent, and dive into Resource Controllers for streamlined CRUD operations. By the end, you'll have a solid understanding of these concepts, along with a practical example demonstrating CRUD operations on a blog post entity.
Difficulty Level: Intermediate
Prerequisites:
- Basic understanding of PHP programming.
- Composer installed on your system.
- Familiarity with Laravel fundamentals.
What is Eloquent ORM?
Laravel's Eloquent ORM (Object-Relational Mapping) is an advanced implementation of the Active Record pattern, allowing developers to work with databases using expressive, object-oriented syntax. It provides an easy-to-use interface for interacting with your database tables, making database operations simple and efficient.
What are Resource Controllers?
Resource Controllers in Laravel are special controllers designed to handle CRUD operations for a particular resource, such as blog posts, users, or products. They provide pre-defined methods for common CRUD operations, reducing boilerplate code and improving code organization.
Step 1: Create a New Laravel Project: Begin by creating a new Laravel project using Composer. Open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel eloquent-resource-example
Step 2: Setup Database: Configure your database settings in the .env file. Set the DB_CONNECTION, DB_HOST, DB_PORT, DB_DATABASE, DB_USERNAME, and DB_PASSWORD variables according to your database configuration.
Step 3: Create Models: Generate a model named Post using Artisan command:
php artisan make: model Post -m
Step 4: Define Database Schema: In the generated migration file (located in database/migrations), define the schema for the posts table with fields such as title, description, category, etc. Here's an example migration file:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description')->nullable();
$table->string('category')->nullable();
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('posts');
}
}
Run the migration using the following Artisan command:
php artisan migrate
Step 5: Create Resource Controller: Generate a Resource Controller named PostController using Artisan command:
php artisan make:controller PostController --resource
Step 6: Define Routes: Define resource routes for the PostController in the routes/web.php file:
use App\Http\Controllers\PostController;
Route::resource('posts', PostController::class);
Step 7: Implement CRUD Operations in Controller: In the PostController, implement methods for CRUD operations using Laravel's Eloquent ORM:
use Illuminate\Http\Request;
use App\Models\Post;
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', ['posts' => $posts]);
}
public function create()
{
return view('posts.create');
}
public function store(Request $request)
{
$request->validate([
'title' => 'required|unique:posts|max:255',
'description' => 'required',
'category' => 'required',
// Add validation rules for other fields
]);
Post::create($request->all());
return redirect()->route('posts.index')->with('success', 'Post created successfully!');
}
public function edit($id)
{
$post = Post::findOrFail($id);
return view('posts.edit', ['post' => $post]);
}
public function update(Request $request, $id)
{
$request->validate([
'title' => 'required|unique:posts|max:255',
'description' => 'required',
'category' => 'required',
// Add validation rules for other fields
]);
Post::findOrFail($id)->update($request->all());
return redirect()->route('posts.index')->with('success', 'Post updated successfully!');
}
public function destroy($id)
{
Post::findOrFail($id)->delete();
return redirect()->route('posts.index')->with('success', 'Post deleted successfully!');
}
}
Step 8: Create Views: Create views for CRUD operations (index.blade.php, create.blade.php, edit.blade.php, show.blade.php) in the resources/views/posts directory.
index.blade.php:
This view displays a list of all blog posts.
<!-- resources/views/posts/index.blade.php -->
@extends('layouts.app')
@section('content')
<h1>Blog Posts</h1>
<a href="{{ route('posts.create') }}" class="btn btn-primary mb-3">Create New Post</a>
<ul class="list-group">
@foreach ($posts as $post)
<li class="list-group-item">
<a href="{{ route('posts.show', $post->id) }}">{{ $post->title }}</a>
</li>
@endforeach
</ul>
@endsection
create.blade.php:
This view contains a form to create a new blog post.
<!-- resources/views/posts/create.blade.php -->
@extends('layouts.app')
@section('content')
<h1>Create New Post</h1>
<form method="POST" action="{{ route('posts.store') }}" enctype="multipart/form-data">
@csrf
<div class="form-group">
<label for="title">Title:</label>
<input type="text" name="title" class="form-control">
</div>
<!-- Add input fields for other attributes such as description, category, etc. -->
<div class="form-group">
<button type="submit" class="btn btn-primary">Create Post</button>
</div>
</form>
@endsection
edit.blade.php:
This view contains a form to edit an existing blog post.
<!-- resources/views/posts/edit.blade.php -->
@extends('layouts.app')
@section('content')
<h1>Edit Post</h1>
<form method="POST" action="{{ route('posts.update', $post->id) }}" enctype="multipart/form-data">
@csrf
@method('PUT')
<div class="form-group">
<label for="title">Title:</label>
<input type="text" name="title" value="{{ $post->title }}" class="form-control">
</div>
<!-- Add input fields for other attributes such as description, category, etc. -->
<div class="form-group">
<button type="submit" class="btn btn-primary">Update Post</button>
</div>
</form>
@endsection
show.blade.php:
This view displays details of a single blog post.
<!-- resources/views/posts/show.blade.php -->
@extends('layouts.app')
@section('content')
<h1>{{ $post->title }}</h1>
<!-- Display other attributes such as description, category, etc. -->
<p>{{ $post->description }}</p>
<p>Category: {{ $post->category }}</p>
<p>Created at: {{ $post->created_at }}</p>
<p>Updated at: {{ $post->updated_at }}</p>
<a href="{{ route('posts.edit', $post->id) }}" class="btn btn-primary">Edit Post</a>
<form method="POST" action="{{ route('posts.destroy', $post->id) }}" style="display: inline-block;">
@csrf
@method('DELETE')
<button type="submit" class="btn btn-danger">Delete Post</button>
</form>
@endsection
Conclusion: Congratulations! You've successfully mastered Laravel Eloquent and Resource Controllers. By following this guide, you've learned how to set up a new Laravel project, define database schema, create models, implement CRUD operations using Resource Controllers, and create views for managing resources. Experiment with different features and functionalities to further enhance your Laravel skills. Happy coding!