Blog Details
Building a REST API with Node.js, Express, and MySQL
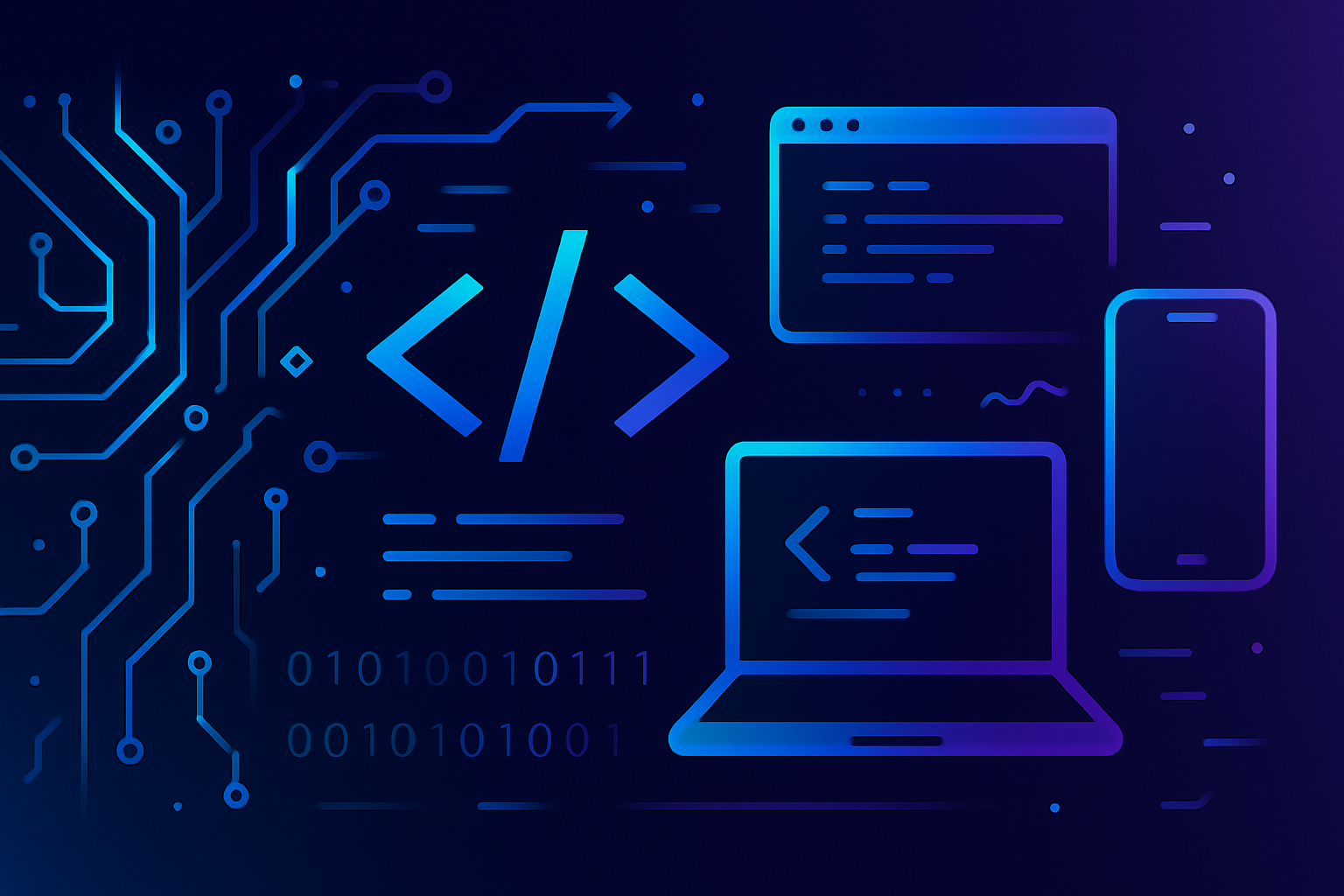
Introduction:
In today's digital landscape, building robust and efficient APIs is essential for developing modern web applications. Among the myriad of technologies available, Node.js stands out as a powerful platform for building scalable and high-performance APIs. In this blog post, we'll delve into the process of creating a REST API using Node.js and Express, backed by a MySQL database. So, let's roll up our sleeves and embark on this exciting journey!
Difficulty Level:
The difficulty level is Intermediate which implies that a basic understanding of programming concepts and familiarity with Node.js, Express, and MySQL is assumed. Coder should be comfortable with handling asynchronous operations, routing, and database interactions within a Node.js environment. While not overly complex, some prior experience or additional research may be necessary to fully grasp the concepts presented in the tutorial.
Prerequisites:
Before we dive into the implementation, ensure you have the following installed on your system:
- Node.js and npm (Node Package Manager)
- MySQL Server
Setting Up Your Project:
- Initialize Your Project: Begin by creating a new directory for your project and navigate into it. Run `npm init` to initialize a new Node.js project. Follow the prompts to create your package.json file.
- Install Dependencies: We'll need Express.js and mysql2 packages for our project. Install them using:
npm install express mysql2
- Setting Up MySQL Database: Create a MySQL database and a table to store your data. You can do this via command-line or a MySQL GUI tool like phpMyAdmin. For example:
CREATE DATABASE mydatabase;
USE mydatabase;
CREATE TABLE items (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL
);
Creating the REST API:
- Initializing Express Server: Create an index.js file in your project directory. Require Express and set up your server.
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
- Connecting to MySQL Database: Add MySQL connection to your index.js file.
const mysql = require('mysql2');
const db = mysql.createConnection({
host: 'localhost',
user: 'root', // Your MySQL username
password: 'password', // Your MySQL password
database: 'mydatabase' // Your MySQL database name
});
db.connect((err) => {
if (err) {
console.error('Error connecting to MySQL database:', err);
return;
}
console.log('Connected to MySQL database');
});
- Implementing CRUD Operations: Define routes to handle CRUD operations (Create, Read, Update, Delete) for your API.
// Get all items
app.get('/items', (req, res) => {
db.query('SELECT * FROM items', (err, results) => {
if (err) {
console.error('Error fetching items:', err);
res.status(500).json({ error: 'Internal server error' });
return;
}
res.json(results);
});
});
// Add a new item
app.post('/items', (req, res) => {
const newItem = req.body;
db.query('INSERT INTO items SET ?', newItem, (err, result) => {
if (err) {
console.error('Error adding item:', err);
res.status(500).json({ error: 'Internal server error' });
return;
}
res.status(201).send('Item added successfully');
});
});
// Update an item
app.put('/items/:id', (req, res) => {
const itemId = req.params.id;
const updatedItem = req.body;
db.query('UPDATE items SET ? WHERE id = ?', [updatedItem, itemId], (err, result) => {
if (err) {
console.error('Error updating item:', err);
res.status(500).json({ error: 'Internal server error' });
return;
}
res.send('Item updated successfully');
});
});
// Delete an item
app.delete('/items/:id', (req, res) => {
const itemId = req.params.id;
db.query('DELETE FROM items WHERE id = ?', itemId, (err, result) => {
if (err) {
console.error('Error deleting item:', err);
res.status(500).json({ error: 'Internal server error' });
return;
}
res.send('Item deleted successfully');
});
});
Conclusion: Congratulations! You've successfully created a simple crud REST API using Node.js, Express, and MySQL. This is just the beginning of your journey into the world of building powerful and scalable web applications. Keep exploring, learning, and innovating. Happy coding!